How to create and render dialog in a web page using jQuery Mobile
In jQuery Mobile, dialogs are very similar to the pages except that they are themed differently to look like a modal dialog. They are generally used to notify user or verify some action the user is about to take. With the help of jQuery Mobile it is very easy to create and display dialog in your mobile web applications.
Here for demonstration purpose we will take up a scenario where user clicks on an external link. Before redirecting the user to the external link we will verify whether he/she really wants to go to the external site by displaying a dialog box. If the user confirms then we redirect the user to the external website otherwise we redirect him/her to the original page.
We will create a multipage template which will contain both our page and dialog i.e. all the code will be present inside a single HTML file only.
Create a new HTML file and add the following code to it:
<!DOCTYPE html> <html> <head> <title>My Page</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="http://code.jquery.com/mobile/1.0.1/jquery.mobile-1.0.1.min.css" /> <script src="http://code.jquery.com/jquery-1.7.1.min.js"></script> <script src="http://code.jquery.com/mobile/1.0.1/jquery.mobile-1.0.1.min.js"></script> </head> <body> <!-- First Page --> <div data-role="page"> <div data-role="header"> <h1>First Page</h1> </div><!-- /header --> <div data-role="content"> <p>Sample page content. Click on the link below to open dialog. </p> <p> <!-- Open the second sub page as a dialog --> <a href="#confirm" data-rel="dialog" data-transition="slidedown"> Go to external link </a> </p> </div><!-- /content --> <div data-role="footer"> <h4>Page Footer</h4> </div><!-- /footer --> </div><!-- /page --> <!-- Dialog page --> <div data-role="page" id="confirm"> <div data-role="header"> <h1>Confirm</h1> </div><!-- /header --> <div data-role="content"> Do you want to go to this external link? <a href="http://www.botskool.com" data-role="button" data-inline="true"> Yes </a> <a href="#" data-role="button" data-inline="true" data-rel="back" data-theme="a"> No </a> </div><!-- /content --> </div><!-- /page --> </body> </html>
Now, open the above HTML file on your mobile device. The main page will look like this as shown below:

If you click on the hyperlink titled Go to external link, then the following dialog box appears:
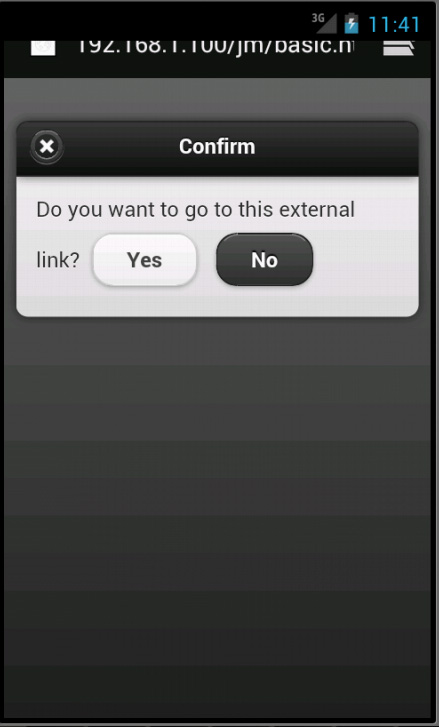
If we click on Yes, then we are taken to the external website (in this case www.botskool.com) otherwise we are taken back to the main page, as shown below:

The complete layout of the dialog has been wrapped inside the div confirm. If you observe closely you will notice that the content of this div is exactly similar to that of a page! It contains header and content sections. Then how does jQuery Mobile determine that this page has to be rendered as a dialog? The trick is to add an additional attribute data-rel to the hyperlink which points to this page/dialog as shown below:
<!-- Open the second sub page as a dialog --> <a href="#confirm" data-rel="dialog" data-transition="slidedown"> Go to external link </a>
Here, the value of the data-rel attribute is dialog which tells the jQuery Mobile to render this link as a dialog. Also, this hyperlink contains another attribute data-transition which determines the type of slide animation played when the dialog is rendered. It can have the following values:
- pop
- slidedown
- flip
When the user clicks on this link the div confirm is rendered as a dialog, as shown below:
<!-- Dialog page --> <div data-role="page" id="confirm"> <div data-role="header"> <h1>Confirm</h1> </div><!-- /header --> <div data-role="content"> Do you want to go to this external link? <a href="http://www.botskool.com" data-role="button" data-inline="true"> Yes </a> <a href="#" data-role="button" data-inline="true" data-rel="back" data-theme="a"> No </a> </div><!-- /content --> </div><!-- /page -->
Here, in the content section we have added two buttons - Yes and No. A hyperlink is rendered as a button if it contains an attribute data-role with value button. The No button contains another attribute named data-rel with value back. This tells the jQuery Mobile to take the user to the previous page when this button is clicked. And, finally if we click on Yes button we are taken to the external website as already discussed above.